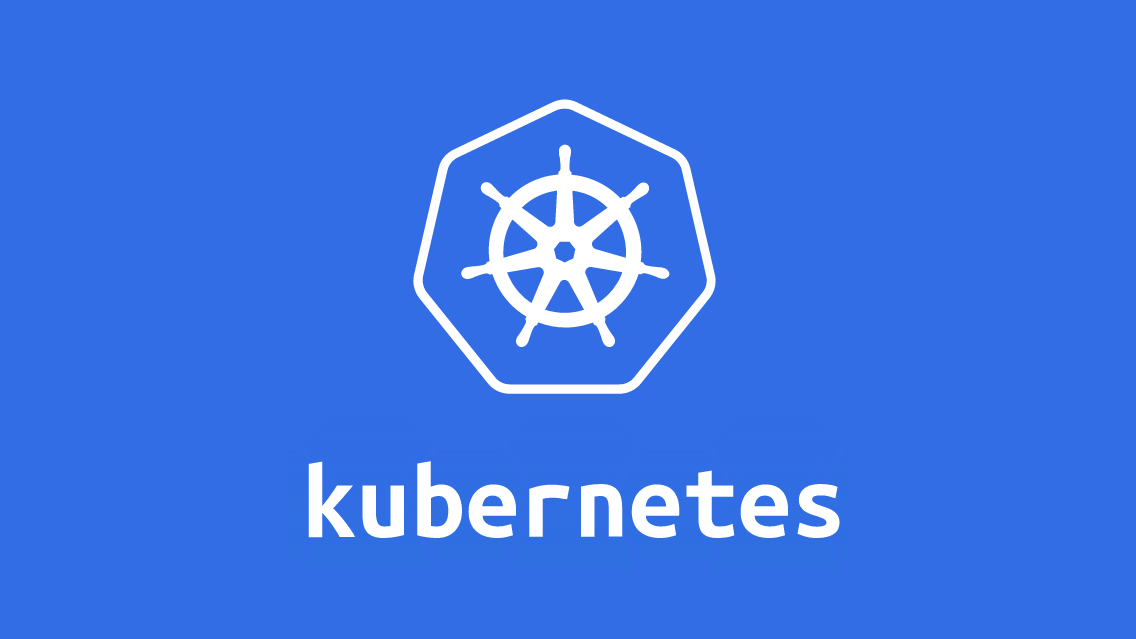
Core Concepts
Cluster Architecture Overview
- Master:
- ETCD cluster: stores information about the cluster in a k-v format.
- Kube-API server: responsible for orchestrating all operations within the cluster
- Kube Controller Manager: takes care of different functions such as node controller (takes care of nodes), replication controller (ensures the desired number of containers are running), etc…
- Kube-scheduler: responsible for scheduling applications or containers on nodes. Basically, it identifies the right node to place the container based on the container resource requirements, the node maximum capacity etc..
- Worker:
- Kubelet: plays the captain role of the ship, takes instructions from the Kube-API server, and manages containers and the kube-proxy.
- kube-proxy: helps in enabling communication between services within the cluster.
- Master:
Kubernetes Control-Plane Components
ETCD
ETCD Overview
- What is ETCD?
- So basically, ETCD is a distributed reliable, and highly available key-value store that is simple, secure & fast.
- ETCD Installation:
- Download the binary
curl -L ${DOWNLOAD_URL}/${ETCD_VER}/etcd-${ETCD_VER}-linux-amd64.tar.gz -o /tmp/etcd-${ETCD_VER}-linux-amd64.tar.gz
- Extract
tar xzvf /tmp/etcd-${ETCD_VER}-linux-amd64.tar.gz -C /usr/bin/etcd --strip-components=1
- RUN ETCD service
etcd --version
⇒ Once the ETCD service runs, it starts to listen on port 2379 by default.
- Download the binary
- Operate ETCD
etcdctl
- change the API version
- M1 for each command:
ETCDCTL_API=3
./etcdctl
version
- M2 for the entire session:
export ETDCTL_API=3
- M1 for each command:
- What is ETCD?
ETCD for Kubernetes
- ETCD stores information about the cluster such as ( nodes, pods, configs, secrets, accounts, roles, bindings, etc..)
Kube-API server
- What is it? ⇒ Primary management component in Kubernetes.
- Responsible for:
- Authenticate user
- Validate request
- Retrieve data
- Update ETCD
- Scheduler
- Kubelet
- The only component that interacts directly with ETCD data store.
Kube Controller Manager
- What is it? ⇒ Responsible for managing various controllers in k8s.
- A controller is a process that continuously monitors the state of various components within the system and works towards bringing them to the desired function state.
- Note Controller:
- Responsible for monitoring the status of the nodes.
- Tests the status of the nodes every 5 seconds through the kube-api server.
- The node is marked unreachable after 40 seconds, giving it 5 minutes to return.
- If it doesn’t, then it will remove all the pods assigned to that node and provision them on the healthy node.
- Replication Controller:
- Responsible for monitoring the status of the replica sets, ensuring that the desired number of pods are available.
- If a pod dies, it creates another one.
- Other Controllers:
- Deployment Controller
- NameSpace Controller
- EndPoint Controller
- Job Controller
- Service Account Controller
- PV-Protection Controller
- CronJob Controller
- Stateful Controller
- PV-Binder Controller
- Note Controller:
Kube Scheduler
- What is it? ⇒ Responsible for scheduling pods on nodes.
- Only decides which pod goes where.
- Uses a scoring system to prioritize the best fit for the container.
Kubelet
- What is it? ⇒ Responsible for managing the nodes.
- They receive instructions from the master nodes.
- Register nodes within the cluster.
- The installation of Kubbelet is done manually for every node.
Kube-proxy
- What is it?
- a process that runs on each node in the k8s cluster to ensure the communication between pods.
- How?
- The kube-proxy job is to look for new services and create the appropriate rules on each node to forward traffic to those services to the backend pods.
- Using
iptable
rules.
- What is it?
Kubernetes workloads Resources
Pods
- What is a Pod?
- Pods are the smallest deployable units of computing that you can create and manage within your Kubernetes cluster. However, A pod consists of a group of one or more tightly related containers that share the same node underlying and the same Linux namespace. and
- How to deploy a Pod?
- Imperative way:
kubectl run nginx --image=nginx:1.14.2 kubectl get pods
- Declarative way:
apiVersion: v1 kind: Pod metadata: name: nginx spec: containers: - name: nginx image: nginx:1.14.2 ports: - containerPort: 80
kubectl create -f nginx_pod.yml kubectl get pods
- Imperative way:
- What is a Pod?
ReplicaSets
- What is a ReplicaSet?
- ReplicaSets are used to maintain a stable set of pods running at any given time. As such, is used to guarantee the availability of a specified number of identical pods.
- How to deploy a ReplicaSet?
apiVersion: apps/v1 kind: ReplicaSet metadata: name: frontend labels: app: guestbook tier: frontend spec: # modify replicas according to your case replicas: 3 selector: matchLabels: tier: frontend template: #Pod template metadata: labels: tier: frontend spec: containers: - name: php-redis image: gcr.io/google_samples/gb-frontend:v3
$ kubectl create -f front-replicas.yml $ kubectl get rs $ kubectl describe rs/frontend
- What is a ReplicaSet?
Deployments
- What is a deployment?
- So basically, Deployments ensure the desired number of pods are running and always available. The update process is also wholly recorded, and versioned with options to pause, continue, and roll back to previous versions.
- The Kubernetes deployment object lets you:
- Deploy a replica set or pod.
- Update pods and replica sets.
- Rollback to the previous version.
- Scale a deployment.
- Pause or continue a deployement.
- How to deploy a deployment?
apiVersion: apps/v1 kind: Deployment metadata: name: nginx-deployment labels: app: nginx spec: replicas: 3 selector: matchLabels: app: nginx template: metadata: labels: app: nginx spec: containers: - name: nginx image: nginx:1.14.2 ports: - containerPort: 80
- What is a deployment?
Services
- What is a Service?
- A service is a method for exposing a network application that is running as one or more Pods in your cluster.
- Why use the Service Kubernetes object?
- To understand the need for a service let’s assume that you are using a Deployment to run your app. that Deploymenet can create and destroy Pods dynamically. Each Pod gets its own IP address, the set of Pods running in one moment in time could be different from the set of Pods running that application a moment later. This leads to a problem; if some set of Pods
Backends
provides functionality to other podsFrontends
inside your cluster, how do the frontends find out and keep track of which IP address to connect to, so that the frontend can use the backend part of the workload? ENTER SERVICES.
- To understand the need for a service let’s assume that you are using a Deployment to run your app. that Deploymenet can create and destroy Pods dynamically. Each Pod gets its own IP address, the set of Pods running in one moment in time could be different from the set of Pods running that application a moment later. This leads to a problem; if some set of Pods
- Service type:
- ClusterIP:
- Exposes the Service on the cluster-internal IP → This will make the Service only reachable from within the cluster.
- NodePort:
- Exposes the Service on each Node’s IP at a static port.
piVersion: v1 kind: Service metadata: name: myapp-service spec: types: NodePort ports: - targetPort: 80 port: 80 nodePort: 30008
- Exposes the Service on each Node’s IP at a static port.
- LoadBalancer:
- Exposes the service externally using an external load balancer. Note that you should provide one as Kubernetes doesn’t directly offer a load-balancing component.
- ExternalName:
- Maps the service to the contents of the
externalName
field (e.g.api.foo.bar.example
). The mapping configures your cluster’s DNS server to return aCNAME
record with that external hostname value.
- Maps the service to the contents of the
- ClusterIP:
- Defining a Service
apiVersion: v1 kind: Service metadata: name: my-service spec: selector: app.kubernetes.io/name: MyApp ports: - protocol: TCP port: 80 targetPort: 9376 #Default Service type is ClusterIP
- What is a Service?
Name Spaces
- What are Namespaces?
- Namespaces is a mechanism for isolating groups of resources within a single cluster.
- Initial namespaces
- default
- kube-node-lease: holds Lease objects associated with each node. Node leases allow the Kubelet to send
heartbeats
so that the control plane can detect node failure.
- kube-public: This namespace is readable by all clients, and is mostly reserved for cluster usage in case some resources should be visible and readable publicly throughout the whole cluster.
- kube-system: used for objects created by the Kubernetes system.
- Working withe namespaces
apiVersion: v1 kind: Namespace metadata: name: mynamespace
$ kubectl create ns <namespace> $ kubectl get ns
- What are Namespaces?