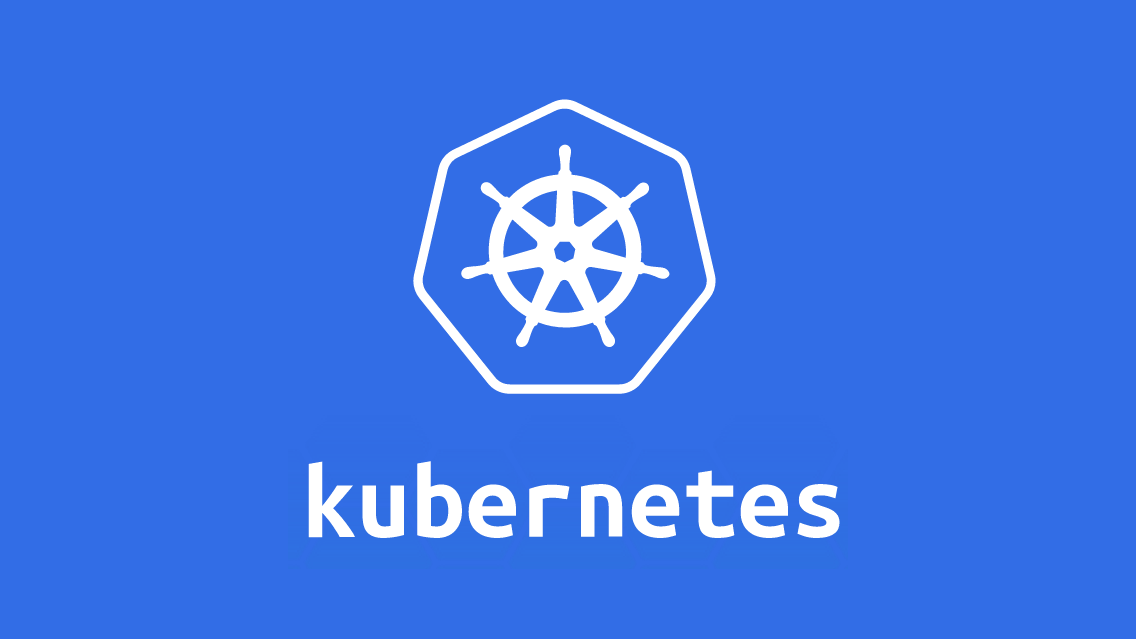
Application LifeCycle Management
Rolling Updates and Rollbacks
- On the deployment creation, a new rollout creates a deployment revision 1. This can help us keep track of the changes made to our deployment and enable us to roll back to the previous version of deployment if necessary.
kubectl rollout status deploy/my-dep
⇒ Check the status of our rollout.
kubectl rollout history deploy/my-dep
⇒ Check the revisions and history of the rollout.
kubectl set image deploy/my-dep nginx=nginx:1.8.1
⇒ Upgrade the container image of your application.
kubectl rollout undo deploy/my-deb
⇒ Roll back to the previous version.
Application Commands & Args
- To override the
ENTRYPOINT
use thecommand
field.
- To override the
CMD
use theargs
field.
apiVersion: v1 kind: Pod metadata: name: my-pod spec: containers: - image: nginx:1.8.1 name: nginx-container command: ["sleep"] args: ["10"]
- To override the
Configure Environment Variables
- Kubernetes allows users to provide env variables when defining Pods.
- You can define your vars under the env field.
apiVersion: v1 kind: Pod metadata: name: my-pod spec: containers: - image: mysql:1.2 name: mysql-container env: - name: MYSQL_USER value: chxmxii
Configure ConfigMaps
- When managing a lot of pods, it will become difficult to manage the env data stored within the definition files. Thus, Kubernetes uses ConfigMaps to manage them centrally.
- ConfigMaps are used to pass configuration data in the form of key-value pairs.
#Imperative way: $ kubectl create configmap <cm_name> --from-literal=<key>=<value> $ kubectl create configmap <cm_name> --from-file=<file_path> #Declartiv approch: apiVersion: v1 kind: ConfigMap metadata: name: <cm_name> data: <key>:<value> ... #working with configmaps $ kubectl create -f . $ kubectl get configmaps $ kubectl describe configmaps #Injecting configmaps in pods apiVersion: v1 kind: Pod metadata: name: myApp spec: containers: - name: nginx-container image: nginx:latest ports: - containerPort: 8080 envFrom: - configMapRef: name: nginx-conf
Configure Secrets
- Sometimes you want to store sensitive information like passwords or keys, But storing them within a configMap is not the best choice since they will exposed in plain text. Kubernetes offers another feature Secrets, Which is similar to ConfigMaps but instead, they are used to store data in an encoded or hashed format.
- Using Secrets is very simple, just like configMaps you just create them and then you inject them within your pod.
#Imperative way $ kubectl create secret <type(usually generic> <secret_name> --from-literal=key=value --from-literal=key1=value1 $ kubectl create secret <type> <secret_name> --from-fime=/path/to/file $ kubectl get secrets $ kubectl describe secrets #Declarative way apiVersion: v1 kind: Secrets metadata: name: <secret_name> type: <secret_type> #e.g. Opaque data: <key>:<value> #e.g value should be b64 encoded password: bXlwYXNzd29yZA== #Inject secret within a pod apiVersion: v1 kind: Pod metadata: name: myPod spec: containers: - image: mysql name: mysql-container envFrom: - secretRef: name: <secret_name> #OR you can call values only. env: - name: MYSQL_PASSWORD valueFrom: secretKeyRef: name: MySQL-secrets key: password
- Type of Secrets
Built-in Type Usage Opaque arbitrary user-defined data kubernetes.io/service-account-token ServiceAccount token kubernetes.io/dockercfg kubernetes.io/dockercfg kubernetes.io/dockerconfigjson serialized ~/.docker/config.json file kubernetes.io/basic-auth credentials for basic authentication kubernetes.io/ssh-auth credentials for SSH authentication kubernetes.io/tls data for a TLS client or server bootstrap.kubernetes.io/token bootstrap token data
Multi Container Pods
- A pod is the smallest component in Kubernetes, It can carry from 1 to ++ containers. those containers will share the same network namespace, shared volumes, and the same life cycle which means containers will be created and destroyed together and can refer to each other as localhost. This way you don’t have to establish volume sharing or services between Pods, to enable communication between them.
apiVersion: v1 kind: Pod metadata: name: my-web-app spec: containers: - name: myapp image: myapp ports: - containerPort: 8080 - name: queue-listener image: queue-listener
- Design Patterns
- Sidecar pattern
- Used to enhance or extend the functionality of the main container.
- e.g. logging utilities, sync services, watchers, and monitoring agents.
- Adaptor pattern
- Used to reformat the output by the primary container in a specific manner that fits the standards across your apps. It acts as a reverse proxy.
- e.g. prometheus-exporter
- Ambassador pattern
- Used to send network requests on behalf of the main application. It acts as a client proxy.
- Sidecar pattern
- A pod is the smallest component in Kubernetes, It can carry from 1 to ++ containers. those containers will share the same network namespace, shared volumes, and the same life cycle which means containers will be created and destroyed together and can refer to each other as localhost. This way you don’t have to establish volume sharing or services between Pods, to enable communication between them.